We can not delete hidden fields directly from sharepoint list forms. In order to delete it, we need to set CanToggleHidden attribute to true & then we can unhide the field & can delete it using Object Model.
Add System.Reflection namespace.
using (SPSite site = new SPSite(site url))
{
using (SPWeb web = site.OpenWeb())
{
SPList testList = web.Lists["Test"];
web.AllowUnsafeUpdates = true;
SPField objTitle = testList.Fields["Title"];
Type type = objTitle.GetType();
MethodInfo mi = type.GetMethod("SetFieldBoolValue",BindingFlags.NonPublic | BindingFlags.Instance);
mi.Invoke(objTitle, new object[] { "CanToggleHidden", true });
objTitle.Hidden = true; //objTitle.Hidden = false;
objTitle.Update();
}
}
Once CanToggleHidden attribute is set to "true" , so you can unhide the field as you wish. and further you can delete the hidden field.
Wednesday, October 27, 2010
Thursday, July 22, 2010
Impersonation in ASP.NET causes [COM.Exception(0x80072020) : An Operations error occurred.]
When you run code that uses DirectorySearcher, DirectoryEntry or other classes that communicates with network resources from a webpart in a Sharepoint site, you recieve a: [COMException (0x80072020): An operations error occurred. ]
This is caused by the fact that when a user is authenticated against a sharepoint server using NTLM or Kerberos, a "secondary token" is sent to the server that it uses to authenticate the user. This token cannot be used to authenticate the current user against another server (e.g. a domain controller).
This can be circumvented by reverting the impersonation to the application pool account used by IIS (if this account has access to Active Directory) with the following code (this is equal to running with impersonation set to false in web.config):
C#:
// This code runs as the application pool user
DirectorySearcher searcher ...
End Using
// Code here runs as logged on user again
This is caused by the fact that when a user is authenticated against a sharepoint server using NTLM or Kerberos, a "secondary token" is sent to the server that it uses to authenticate the user. This token cannot be used to authenticate the current user against another server (e.g. a domain controller).
This can be circumvented by reverting the impersonation to the application pool account used by IIS (if this account has access to Active Directory) with the following code (this is equal to running with impersonation set to false in web.config):
C#:
using System.Web.Hosting;
...
...
...
...
// Code here runs as the logged on user
using (HostingEnvironment.Impersonate()) {// This code runs as the application pool user
DirectorySearcher searcher ...}
// Code here runs as logged on user again
using (HostingEnvironment.Impersonate()) {// This code runs as the application pool user
DirectorySearcher searcher ...}
// Code here runs as logged on user again
VB:
// Code here runs as the logged on user
Using (System.Web.Hosting.HostingEnvironment.Impersonate())
Using (System.Web.Hosting.HostingEnvironment.Impersonate())
// This code runs as the application pool user
DirectorySearcher searcher ...
End Using
// Code here runs as logged on user again
Monday, June 21, 2010
Writing Messages to Event Log in SharePoint
In SharePoint using System.Diagnostics.EventLog class, you can write messages to event log.
Below is the code snippet for the same,
string source = "Demo Application";
if (!EventLog.SourceExists(source))
EventLog.CreateEventSource(source, "Application");
EventLog.WriteEntry(source, "Message!", EventLogEntryType.Error);
Below is the code snippet for the same,
string source = "Demo Application";
if (!EventLog.SourceExists(source))
EventLog.CreateEventSource(source, "Application");
EventLog.WriteEntry(source, "Message!", EventLogEntryType.Error);
Thursday, June 17, 2010
Custom aspx pages in SharePoint 2010
While migrating Custom aspx pages from MOSS 2007 to SP 2010, below mentioned pages directives are to be modified accordingly.
MOSS way of doing
<%@ Page Language="C#" Inherits="Microsoft.SharePoint.ApplicationPages.NewListPage" MasterPageFile="~/_layouts/application_Custom.master" %>
SP 2010 way of declaring the directive,
<%@ Page language="C#"
MasterPageFile= "~/_layouts/app lication_Custom.master"
Inherits="Micro soft.SharePoint .WebPartPages.W ebPartPage,Micr osoft.SharePoin t,Version=14.0. 0.0,Culture=neu tral,PublicKeyT oken=71e9bce111 e9429c"%>
MasterPageFile=
Inherits="Micro
If the page inherits publishing layouts page, the directive should be modified as shown below,
MOSS way of doing:
<%@ Page Language="C#" DynamicMasterPageFile="~ masterurl/default.master" Inherits="Microsoft. SharePoint.Publishing. Internal.CodeBehind. CategoriesPage"%>
SP 2010 way of doing:
Follow the below two steps to apply master page here. MasterPageFile tag is not supported directly.
1. <%@ Page Language="C#" Inherits="Microsoft. SharePoint.Publishing. PublishingLayoutPage, Microsoft.SharePoint,Version= 14.0.0.0,Culture=neutral, PublicKeyToken= 71e9bce111e9429c"%>
2. And, then under script tag, override "OnPreInit" method & assign master page there.
protected override void OnPreInit(EventArgs e){
base.OnPreInit(e);
this.MasterPageFile = "SomeOther.master";
}
Monday, June 14, 2010
Copy attachments from one list item to another within a SharePoint site
Attachments for a list item are stored as SPFile objects under a hidden folder in the list where those attachments are stored. Each list item that has an attachment has its own folder with the ID of the item being the folder's name. Here is a code sample to copy attachments from one item to another:
private void CopyAttachments(SPWeb oSPWeb, SPListItem sourceItem, SPListItem targetItem)
{
//get the folder with the attachments for the source item
SPFolder sourceItemAttachmentsFolder =
sourceItem.Web.Folders["Lists"].SubFolders[sourceItem.ParentList.Title]
.SubFolders["Attachments"].SubFolders[sourceItem.ID.ToString()];
//Loop over the attachments, and add them to the target item
foreach (SPFile file in sourceItemAttachmentsFolder.Files)
{
byte[] binFile = file.OpenBinary();
oSPWeb.AllowUnsafeUpdates=true;
targetItem.Attachments.AddNow(file.Name, binFile);
oSPWeb.AllowUnsafeUpdates=false;
}
}
private void CopyAttachments(SPWeb oSPWeb, SPListItem sourceItem, SPListItem targetItem)
{
//get the folder with the attachments for the source item
SPFolder sourceItemAttachmentsFolder =
sourceItem.Web.Folders["Lists"].SubFolders[sourceItem.ParentList.Title]
.SubFolders["Attachments"].SubFolders[sourceItem.ID.ToString()];
//Loop over the attachments, and add them to the target item
foreach (SPFile file in sourceItemAttachmentsFolder.Files)
{
byte[] binFile = file.OpenBinary();
oSPWeb.AllowUnsafeUpdates=true;
targetItem.Attachments.AddNow(file.Name, binFile);
oSPWeb.AllowUnsafeUpdates=false;
}
}
Friday, June 4, 2010
SharePoint people picker is not able to resolve names from different forests / domains
Some time back, I was caught into this issue after upgrading MOSS from SP1 to SP2. People Picker wasn't able to resolve users from different forests / domains.
After struggling for plenty of hours, I got the solution. Actually when you have to fetch users from different forests or domains, you should have user id & password detail of that specific domain with read access to directory services of that AD.
Execute the below mentioned command to edit property of people picker so that it can search cross forest / domain users.
Step 1. stsadm -o setapppassword -password key
Step 2. stsadm -o setproperty -url "http://portal_URL" -pn peoplepicker-searchadforests -pv "forest:FQFN;domain:FQDN",LoginID,Password
Step 1= Encrypts the password
Step 2= sets property of people picker.
a. portal_URL = Specify your portal / application URL
b. FQFN = Fully Qualified Forest Name
c. FQDN = Fully Qualified Domain Name
d. Login ID = Specify Login ID as (Domain Name\xyz)
e. Password = Specify password for the login. If password contains any special character, provide the password details in double chords. e.g "Hello,11"
If you have to add multiple forests / domains to people picker, you may have to execute below mentioned command for the same.
Step 1: stsadm -o setapppassword -password key
Step 2. stsadm -o setproperty -url "http://portal_URL" -pn peoplepicker-searchadforests -pv "forest:FQFN1;domain:FQDN1",LoginID1,Password1;"forest:FQFN2;
domain:FQDN2",LoginID2,Password2
As shown above, you can add multiple domains in single command line.
FQFN1, FQDN1, LoginID1, Password1 = details for first forest / domain
FQFN2, FQDN2, LoginID2, Password2 = details for second forest / domain
Note: Login IDs should atleast have read access to directory services of respective ADs.
Hope this may help you. Happy Blogging !!!
After struggling for plenty of hours, I got the solution. Actually when you have to fetch users from different forests or domains, you should have user id & password detail of that specific domain with read access to directory services of that AD.
Execute the below mentioned command to edit property of people picker so that it can search cross forest / domain users.
Step 1. stsadm -o setapppassword -password key
Step 2. stsadm -o setproperty -url "http://portal_URL" -pn peoplepicker-searchadforests -pv "forest:FQFN;domain:FQDN",LoginID,Password
Step 1= Encrypts the password
Step 2= sets property of people picker.
a. portal_URL = Specify your portal / application URL
b. FQFN = Fully Qualified Forest Name
c. FQDN = Fully Qualified Domain Name
d. Login ID = Specify Login ID as (Domain Name\xyz)
e. Password = Specify password for the login. If password contains any special character, provide the password details in double chords. e.g "Hello,11"
If you have to add multiple forests / domains to people picker, you may have to execute below mentioned command for the same.
Step 1: stsadm -o setapppassword -password key
Step 2. stsadm -o setproperty -url "http://portal_URL" -pn peoplepicker-searchadforests -pv "forest:FQFN1;domain:FQDN1",LoginID1,Password1;"forest:FQFN2;
domain:FQDN2",LoginID2,Password2
As shown above, you can add multiple domains in single command line.
FQFN1, FQDN1, LoginID1, Password1 = details for first forest / domain
FQFN2, FQDN2, LoginID2, Password2 = details for second forest / domain
Note: Login IDs should atleast have read access to directory services of respective ADs.
Hope this may help you. Happy Blogging !!!
Thursday, May 27, 2010
Stsadm to Windows PowerShell mapping (SharePoint Server 2010)
Microsoft has published a very useful new article on Technet that lists STSADM operations and their equivalent PowerShell cmdlets. Check this link.
Thursday, May 13, 2010
Disable event firing in sharepoint when updating list item
Below piece of code can be used to update SharePoint list item inside any event receiver. I have written it for Updated event receiver.
public override void ItemUpdated(SPItemEventProperties properties)
{
//some handling here
ListItem item = properties.ListItem;
item["someproperty"] = "some value";
this.DisableEventFiring();
//save changes added
Item.Update();
this.EnableEventFiring();
}
Monday, April 5, 2010
Microsoft Tech.Ed 2010, India: In Bangalore from April 12-14
Yes, Its time to pack our bags for TechEd 2010. I am excited & will be attending the largest technology conference globally this year. This time, it is held up in Bangalore from 12th April to 14th April.
I have got the registration in development track & I am keen interested in watching out SharePoint track there.
I am looking forward to TechEd 2010. Hurray :-)

*Picture taken from TechEd 2010 India Site for Blogging PurposeI have got the registration in development track & I am keen interested in watching out SharePoint track there.
I am looking forward to TechEd 2010. Hurray :-)

For more information visit http://www.microsoftteched.in
Monday, March 29, 2010
Upgrade Checker in SharePoint SP2 – prepare your way to SharePoint Server 2010
The upgrade checker in MOSS/WSS SP2 stsadm operation is very useful. It checks server farm for system requirements, database health and a list of rules. The rules can also be extended.
To use upgrade checker, first open a command line prompt, and run
"X:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\12\BIN\STSADM.EXE" -o preupgradecheck
(X is the drive letter where you install SharePoint)
Make sure you are in administrator mode. Otherwise it would be denied.
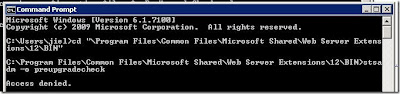
You can see there’re a list of rules checked by the operation.
SearchContentSourcesInfo
SearchInfo
ServerInfo
FarmInfo
UpgradeTypes
SiteDefinitionInfo
LanguagePackInfo
FeatureInfo
AamUrls
LargeList
CustomListViewInfo
CustomFieldTypeInfo
CustomWorkflowActionsFileInfo
ModifiedWebConfigWorkflowAuthorizedTypesInfo
ModifiedWorkflowActionsFileInfo
DisabledWorkFlowsInfo
OSPrerequisite
WindowsInternalDatabaseMigration
WindowsInternalDatabaseSite
MissingWebConfig
ReadOnlyDatabase
InvalidDatabaseSchema
ContentOrphan
SiteOrphan
PendingUpgrade
InvalidServiceAccount
InvalidHostName
A successful run could show the following:
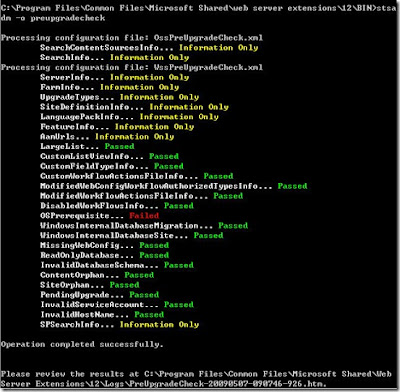
Hey, we got a “OSPrerequisite… Failed” here. So let’s take a look at the report.
The report will give you the following information:
Search content sources and start addresses
Office Server Search topology information
Servers in the current farm
The components from this farm
Supported upgrade types
Site Definition Information
Language pack information
Feature Information
Alternate Access Mapping Url(s) within the current environment that should be considered when upgrading.
Lists and Libraries
Customized field types that will not be upgraded
Windows SharePoint Services Search topology information
And also the failed items it checked.
In my case, because this machine is still on Windows Server 2003 32bit, so it does not meet the requirement of SharePoint Server 2010, which needs to be install on Windows Server 2008 x64.
Failed : This server machine in the farm does not have Windows Server 2008 or higher 64 bit edition installed.
Upgrading to Windows SharePoint Services 4.0 requires Windows Server 2008 or higher 64 bit edition.
Please upgrade the server machines in your farm to Windows Server 2008 64 bit edition, or create a new farm and attach the content from this farm. For more information about this rule, see KB article 954770 in the rule article list at http://go.microsoft.com/fwlink/?LinkID=120257.
To use upgrade checker, first open a command line prompt, and run
"X:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\12\BIN\STSADM.EXE" -o preupgradecheck
(X is the drive letter where you install SharePoint)
Make sure you are in administrator mode. Otherwise it would be denied.
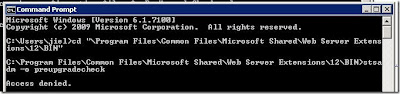
You can see there’re a list of rules checked by the operation.
SearchContentSourcesInfo
SearchInfo
ServerInfo
FarmInfo
UpgradeTypes
SiteDefinitionInfo
LanguagePackInfo
FeatureInfo
AamUrls
LargeList
CustomListViewInfo
CustomFieldTypeInfo
CustomWorkflowActionsFileInfo
ModifiedWebConfigWorkflowAuthorizedTypesInfo
ModifiedWorkflowActionsFileInfo
DisabledWorkFlowsInfo
OSPrerequisite
WindowsInternalDatabaseMigration
WindowsInternalDatabaseSite
MissingWebConfig
ReadOnlyDatabase
InvalidDatabaseSchema
ContentOrphan
SiteOrphan
PendingUpgrade
InvalidServiceAccount
InvalidHostName
A successful run could show the following:
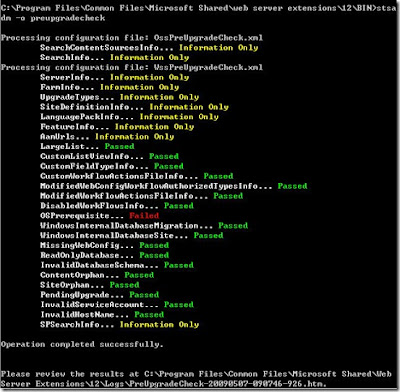
Hey, we got a “OSPrerequisite… Failed” here. So let’s take a look at the report.
The report will give you the following information:
Search content sources and start addresses
Office Server Search topology information
Servers in the current farm
The components from this farm
Supported upgrade types
Site Definition Information
Language pack information
Feature Information
Alternate Access Mapping Url(s) within the current environment that should be considered when upgrading.
Lists and Libraries
Customized field types that will not be upgraded
Windows SharePoint Services Search topology information
And also the failed items it checked.
In my case, because this machine is still on Windows Server 2003 32bit, so it does not meet the requirement of SharePoint Server 2010, which needs to be install on Windows Server 2008 x64.
Failed : This server machine in the farm does not have Windows Server 2008 or higher 64 bit edition installed.
Upgrading to Windows SharePoint Services 4.0 requires Windows Server 2008 or higher 64 bit edition.
Please upgrade the server machines in your farm to Windows Server 2008 64 bit edition, or create a new farm and attach the content from this farm. For more information about this rule, see KB article 954770 in the rule article list at http://go.microsoft.com/fwlink/?LinkID=120257.
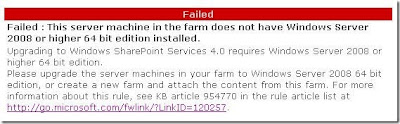
Subscribe to:
Posts (Atom)